On MERN Social, each user will see posts that have been shared by people they follow, along with posts that they themselves share, all aggregated in a Newsfeed view. Before delving further into the implementations of the post-related features in MERN Social, we will look at the composition of this Newsfeed view to showcase a basic example of how to design nested UI components that share state. The Newsfeed component will contain two main child components – a new post form and a list of posts from followed users, as shown in the following screenshot:
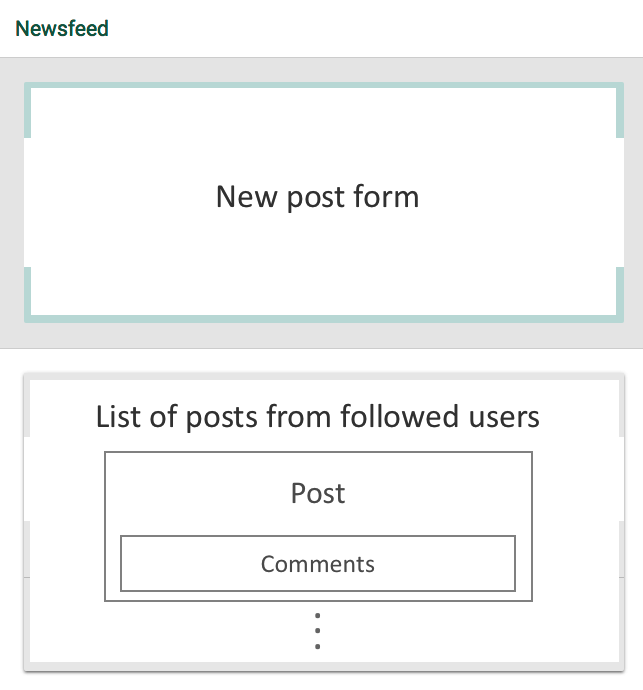
The basic structure of the Newsfeed component will be as follows, with the NewPost component and the PostList component inside it.
mern-social/client/post/Newsfeed.js:
<Card>
<Typography type="title"> Newsfeed </Typography>
<Divider/>
<NewPost addUpdate={addPost}/>
<Divider/>
<PostList removeUpdate={removePost} posts={posts}/>
</Card>
As the parent component, Newsfeed will control the state of the posts' data that's rendered in the child components. It will provide a way to update the state of posts across the components when the post data is modified within the child components, such as the addition of a new post in the NewPost component or the removal of a post from the PostList component.
Here specifically, in the Newsfeed component we initially make a call to the server to fetch a list of posts from people that the currently signed-in user follows. Then we set this list of posts to the state to be rendered in the PostList component.. The Newsfeed component provides the addPost and removePost functions to NewPost and PostList, which will be used when a new post is created or an existing post is deleted to update the list of posts in the Newsfeed's state and ultimately reflect it in the PostList.
The addPost function defined in the Newsfeed component will take the new post that was created in the NewPost component and add it to the posts in the state. The addPost function will look as follows.
mern-social/client/post/Newsfeed.js:
const addPost = (post) => {
const updatedPosts = [...posts]
updatedPosts.unshift(post)
setPosts(updatedPosts)
}
The removePost function defined in the Newsfeed component will take the deleted post from the Post component in PostList and remove it from the posts in the state. The removePost function will look as follows.
mern-social/client/post/Newsfeed.js:
const removePost = (post) => {
const updatedPosts = [...posts]
const index = updatedPosts.indexOf(post)
updatedPosts.splice(index, 1)
setPosts(updatedPosts)
}
As the posts are updated in the Newsfeed's state this way, the PostList will render the changed list of posts to the viewer. This mechanism of relaying state updates from parent to child components and back will be applied across other features, such as comment updates in a post and when a PostList is rendered for an individual user in the Profile component.
To begin the complete implementation of the Newsfeed, we need to be able to fetch a list of posts from the server and display it in the PostList. In the next section, we will make this PostList component for the frontend and add PostList API endpoints to the backend.