An aspect of generics is the determination of what T is and therefore how we can handle it. In C#, we can use System.Reflection and use the GetType method to find the type or use typeof when comparing types.
In Rust, we use std::any:Any. This is a type to emulate dynamic typing:
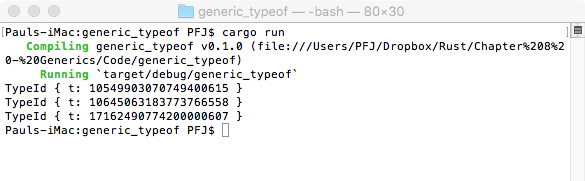
Just by looking at this output, you may be thinking: What on earth are those numbers? I expected something like f32.
What we're seeing here is the ID for the type rather than the type. To actually show the variable type, we have do something slightly different:
#![feature(core_intrinsics)]
fn display_type<T>(_: &T)
{
let typename = unsafe {std::intrinsics::type_name::<T>()};
println!("{}", typename);
}
fn main()
{
display_type(&3.14f32);
display_type(&1i32);
display_type(&1.555);
display_type(&(vec!(1,3,5)));
}
When the preceding code is run on the Rust Playground website, the following results are obtained:

While most of the code we have seen many times, we have not yet come across unsafe and the shebang (#!) in the code.